Level Editor
In this project I created a program that mimics what scene editors in game engines do. The goal of this project was to get basic functionality of game objects and be able to attach various components onto them. This project uses C++, the raylib library, and ImGui.
Game Objects & Components
In the scene we are spawning in gameobjects, but just an empty gameobject won’t do anything. That's why we need to add components onto the game object. Game Objects always need a renderer and a transform (position) to exist in a scene, but there are other components for additional functionality such as a player controller and colliders.
Editing Game Objects
To edit our game objects we need to be able to select them. For this to function we need to get the mouse’s position and compared it to the colliders of our game objects to select which game object is the one we are clicking on.
Combining this with ImGui UI and our components we are able to create a menu that can edit all aspects of our game objects.
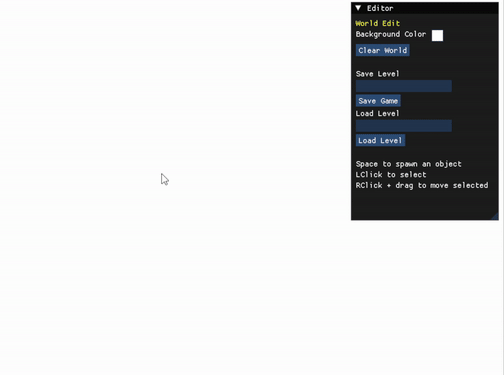
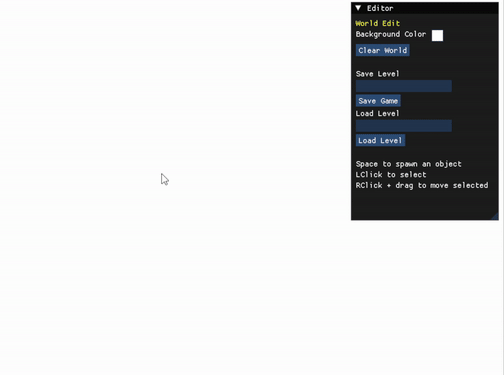
Save & Load
After we create our levels we want to be able to save them for later. Using a text file we are able to convert all game objects and components into text that can be read by our program to create the exact same scene as when we saved it. The text file needs to use a specific format.
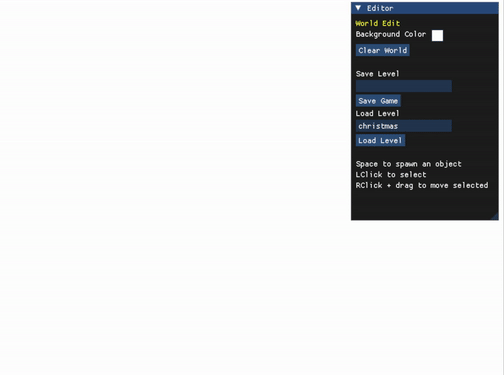
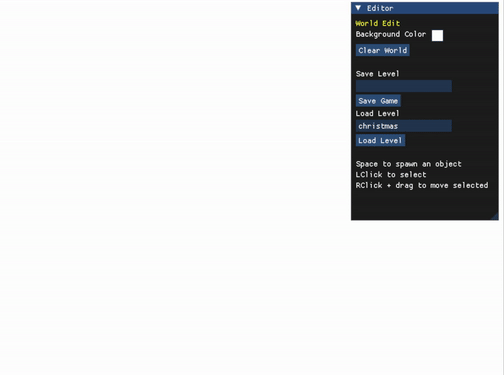
After we create our levels we want to be able to save them for later. Using a text file we are able to convert all game objects and components into text that can be read by our program to create the exact same scene as when we saved it. Here's some of the code for both functions
These functions will read in and output the text into a specific format. This is an example of a file that the code will create and can read to save and load levels.
Component 0 is the transform, or the position of the game object. Component 1 is the renderer which contains the size and color of the game object. Component 2 is the player controller and will allow you to move this game object around the level. Component 3 is the collider component which will detects collision with other game objects with this same component. Finally is component 4 which will change the color of the game object when it detects a collision.